Managing Secrets in Modelbit
You can store secrets privately and securely in Modelbit so that they are never in your code, data or logs. This is especially useful for API keys like your OpenAI API keys as well as AWS Access Keypairs.
Storing Secrets
Go to settings and click the "Secrets" tab in the left-hand navigation. From here, click the "Add Secrets" button to add a secret with the name and secret value.
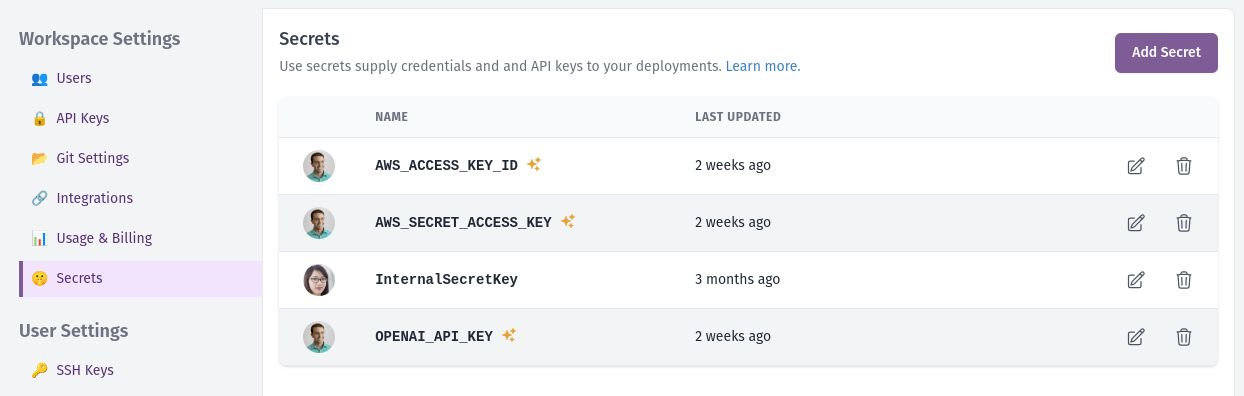
Accessing Secrets
You can access secrets using mb.get_secret
.
For example, to use a secret with your OpenAI API Key:
# First login to Modelbit
import modelbit as mb
import openai
def chat_with_chatgpt(prompt: str) -> str:
openai.api_key = mb.get_secret("OPENAI_API_KEY")
completion = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[{"role": "user", "content": prompt}]
)
return completion.choices[0].message.content