log_image
Log a matplotlib Figure, PIL Image, or path to a png
/jpg
image to Modelbit.
The image will show in the logs viewer of the deployment or training job.
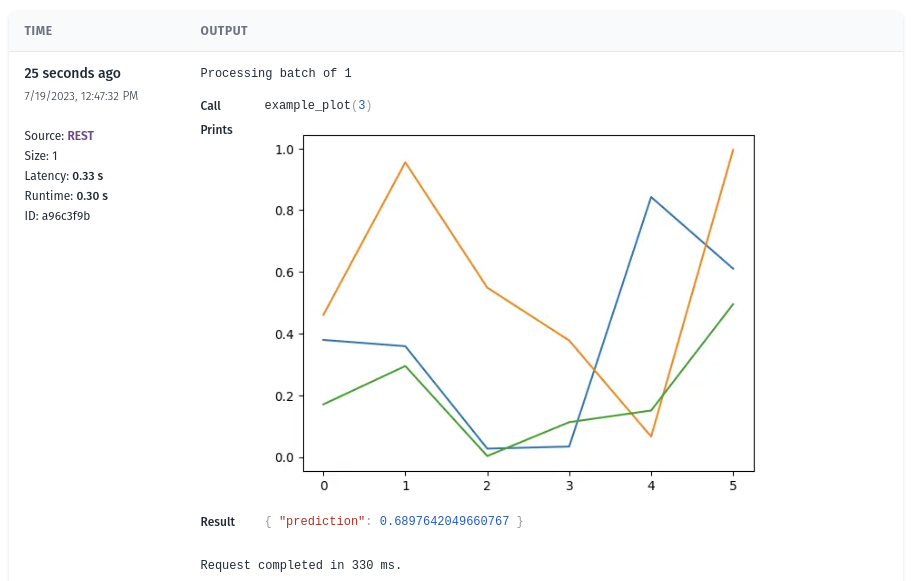
Parameters
mb.log_image({image})
image
:[matplotlib.pyplot.Figure | PIL.Image | str]
This argument can be a matplotlib Figure, a PIL Image, or a string filepath to a local.png
or.jpg
image.
Returns
None
Outside of a Modelbit deployment, calling log_image
prints a success message if it's able to process the supplied image. If the image argument cannot be processed for logging an exception is raised.
Example
Logging a matplotlib Figure
When logging a matploylib
image, pass the figure
to log_image
:
import matplotlib.pyplot as plt
from random import random
def example_plot():
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [random(), random(), random(), random()])
mb.log_image(fig)
return { "prediction": 1 }
Logging the default matplotlib Figure
When you run plt.plot
and related API calls directly rather than on subplots, pass plt.gcf()
as the argument to mb.log_image
:
import matplotlib.pyplot as plt
def example_plot():
plt.plot([1, 2, 3, 4], [random(), random(), random(), random()])
mb.log_image(plt.gcf())
return { "prediction": 1 }
Logging a PIL Image
Pass an Image
instance when using PIL:
from PIL import Image
def example_plot():
img = Image.open("my_image.jpg")
mb.log_image(img)
return { "prediction": 1 }
Logging an image file
When your image is stored locally as a png
or jpg
, pass the path to that file to log_image
:
def example_plot():
mb.log_image("my_image.jpg")
return { "prediction": 1 }
Logging multiple images
To log multiple images, call log_image
multiple times:
import matplotlib.pyplot as plt
def example_plot():
fig, ax = plt.subplots()
print("Before:")
ax.plot([1, 2, 3, 4], [random(), random(), random(), random()])
mb.log_image(fig)
print("After:")
ax.plot([1, 2, 3, 4], [random(), random(), random(), random()])
mb.log_image(fig)
return { "prediction": 1 }